Custom Class
Custom class
Put a lua file of your own class in commompinets/custom folder
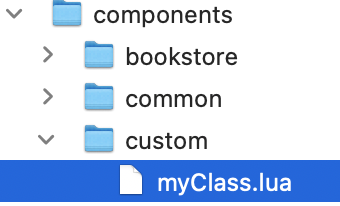
Set your classname to a layer in App/bookX/pageX/index.lua
local sceneName = ...
--
local scene = require('controller.scene').new(sceneName, {
name = "page1",
components = {
layers = {
{ bg={ } },
{ gotoBtn={ } },
{ title={ class={"myClass"} } },
},
audios = {},
groups = {},
timers = {},
variables = {},
page = { }
},
commands = { },
onInit = function(scene) print("onInit") end
})
--
return scene
myClass.lua will be called with a display image object of a layer associated with
local yourLibrary = require("lib.yourLibrary")
--
--
local M = {}
--
function M:init(UI)
end
--
function M:create(UI)
local obj = UI.sceneGroup[self.name]
end
--
function M:didShow(UI)
end
--
function M:didHide(UI)
end
--
function M:destroy(UI)
end
M.set = function(instance)
return setmetatable(instance, {__index=M})
end
--
return M
properties can be defined in App/bookX/pageX/components/layers/layerX_myClass.lua
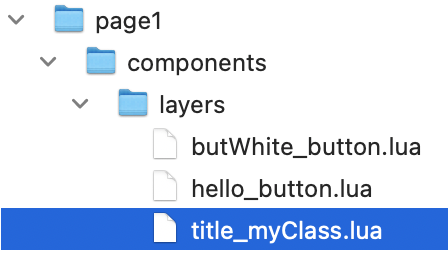
M.params={
mytext = "hello my class",
}
...
...
return M
class as a name space
you can use a classname as name space
For instance, transition2.lua is a custom class and it has functions like “to”, “from”, “moveBy” etc.
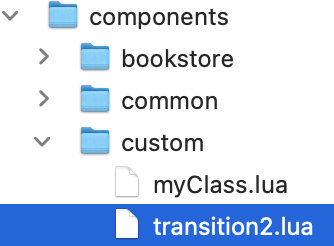
you can speficy a function name as classoption with “.” in App/bookX/components/pageX/index.lua
syntax is className.classOption
local sceneName = ... -- local scene = require('controller.scene').new(sceneName, { name = "page1", components = { layers = { { title={ class={"transition2.to"} } }, }, ... ... }) -- return scene
title layer now uses transition2 class, you can create your own class and write codes in components/common folder. For instance,
components/custom/transition2.lua
classOption contains “to”
local parent,root, M = newModule(...) -- local transition2 = require("extlib.transition2") local _layerProps = { name = M.name, text = "hello my class", } -- function M:init(UI) end -- function M:create(UI) end -- function M:didShow(UI) local classOption = self.classOption or "to" local obj = UI.sceneGroup[self.name] if obj then self.transition_id = transition2[classOption](obj, self.params or {x=10}) end end -- function M:didHide(UI) --transtiion2.cancel(self.transition_id) end -- function M:destroy(UI) end -- function M:new(props) return self:newInstance(props, _layerProps) end -- return M
self.params for transition2.lua should be defined in in App/bookX/pageX/layers/title_transiton2.lua
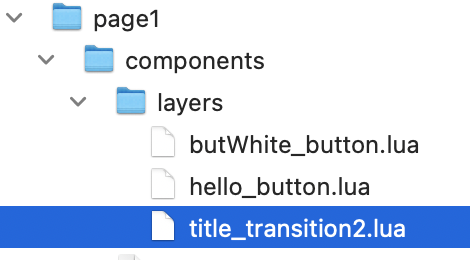
M.params ={
{x = 260, delay = 400},
{y = 260, xScale = -0.8, delay = 800},
{xScale = -1, yScale = 1, delay = 400},
{x = 60, xScale = -0.6, yScale = 0.6, delay = 1200},
{rotation = 135, delay = 400},
{x = 260, y = 60, alpha = 0, delay = 400},
{rotation = 0, alpha = 1, delay = 800},
{x = 60, delay = 400},
{xScale = 0.8, yScale = 0.8, delay = 400}
}
for imported class
use classOption with an opion value for instance “to” for trasition2 in M.props
local M={
path = "bookTest.components.page2.layers.three.index",
name = "three",
}
M.props = {
five_transition2 = {classOption="to"},
}
return require("components.kwik.importer").new(M)
Generic Editor
ToDo
- Add custom class button to the toolbar
- show a list of available custom classes from lfs in components/custom folder
- selectLayer to list custom classes as well
- select a custom class name to show popsTable for editing
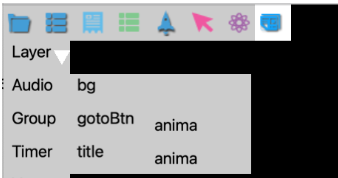
REST httpYac
### selectLayer with class if exists or not
GET /bookFree/page1/title/?class=transition2.to
###
POST /bookFree/page1/title/?class=transition2.to
Content-Type: application/lua
{
{x = 260, delay = 400},
{y = 260, xScale = -0.8, delay = 800},
{xScale = -1, yScale = 1, delay = 400},
{x = 60, xScale = -0.6, yScale = 0.6, delay = 1200},
{rotation = 135, delay = 400},
{x = 260, y = 60, alpha = 0, delay = 400},
{rotation = 0, alpha = 1, delay = 800},
{x = 60, delay = 400},
{xScale = 0.8, yScale = 0.8, delay = 400}
}