Eagle API
Eagle API
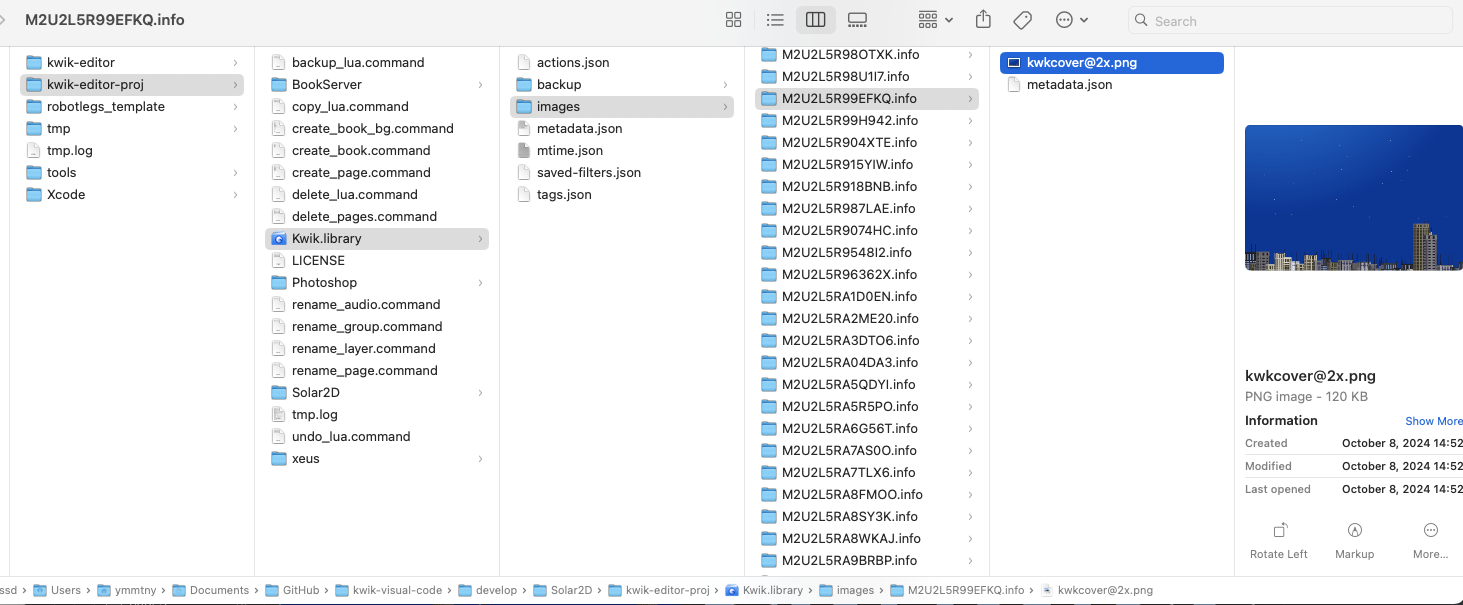
To export a file from the Eagle library to your folder using the Eagle API, you can follow these steps:
- First, you need to retrieve the file you want to export from the Eagle library.
- Then, use the API to get the file’s path and other necessary information.
- Finally, use Node.js file system operations to copy the file to your desired folder.
Here’s a more detailed explanation of how to accomplish this:
Retrieving the File
Use the eagle.item.getSelected()
method to get the currently selected item in the Eagle library[1]. This method returns an array of selected items.
Getting File Information
Once you have the selected item, you can access its properties, including the file path. The src
property of the item object contains the file path[1].
Exporting the File
To export the file, you’ll need to use Node.js fs
(file system) module to copy the file from its original location to your desired folder.
Here’s a code snippet that demonstrates this process:
const fs = require('fs');
const path = require('path');
// Get the selected item
eagle.item.getSelected().then(items => {
if (items.length > 0) {
const item = items[0];
const sourcePath = item.src;
const fileName = path.basename(sourcePath);
const destinationPath = '/path/to/your/folder/' + fileName;
// Copy the file
fs.copyFile(sourcePath, destinationPath, (err) => {
if (err) {
console.error('Error exporting file:', err);
} else {
console.log('File exported successfully');
}
});
} else {
console.log('No item selected');
}
}).catch(err => {
console.error('Error getting selected item:', err);
});
In this code:
- We use
eagle.item.getSelected()
to get the selected item. - We extract the source path (
item.src
) and the file name. - We construct the destination path where we want to export the file.
- We use
fs.copyFile()
to copy the file from the source to the destination.
Remember to replace ‘/path/to/your/folder/’ with the actual path where you want to export the file.
This approach allows you to export a file from the Eagle library to any folder on your system using the Eagle Plugin API and Node.js file system operations[1].
Citations: [1] https://developer.eagle.cool/plugin-api